Full Stack Software Engineering for Beginners
June 20th, 2023
Introduction to software engineering and full stack development
Software engineering is a discipline that encompasses the systematic design, development, and maintenance of software systems. It involves applying engineering principles and practices to create reliable, efficient, and scalable software solutions.
Full stack development refers to the skill set required to develop both the front-end and back-end components of a web application.
A full stack developer is proficient in multiple programming languages and frameworks, enabling them to work on all layers of the software stack, from the user interface to the server-side logic and database integration. This holistic approach allows full stack developers to understand the entire software development process and build end-to-end solutions that meet user requirements.
Frontend vs. Backend
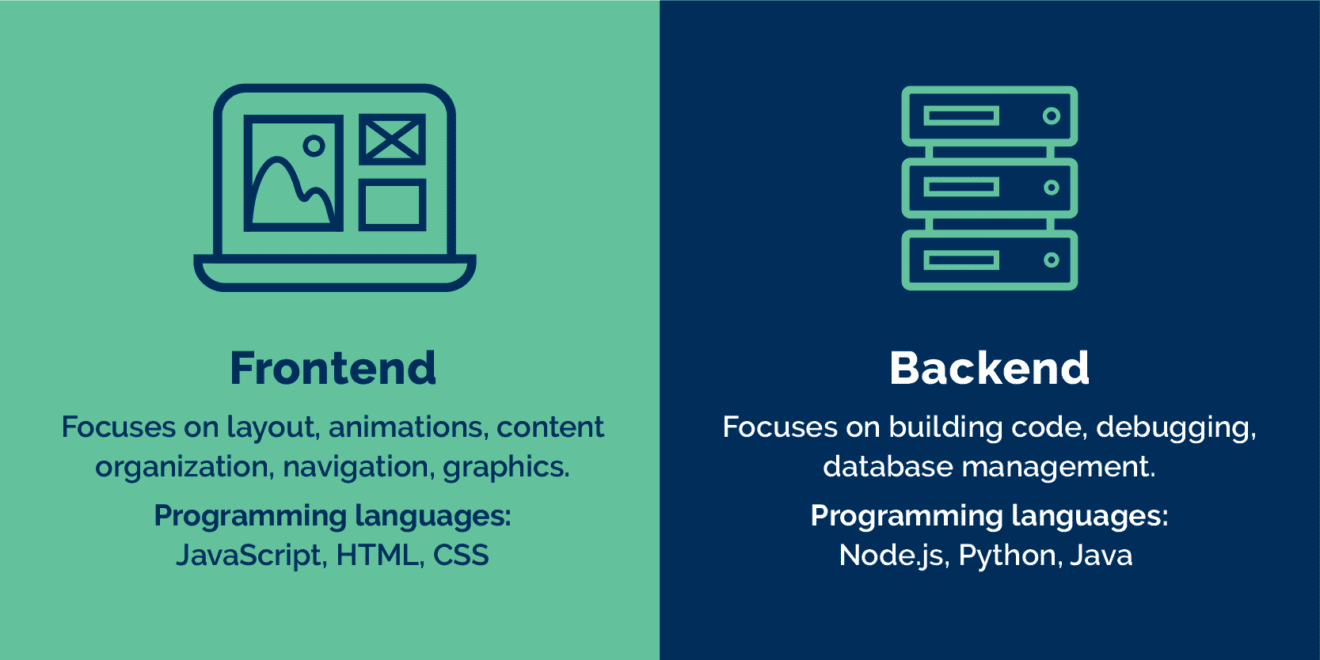
Frontend development focuses on the user interface and user experience (UI/UX) aspects of an application. It involves creating the visual elements and interactive features that users interact with directly. Frontend developers use technologies like HTML, CSS, and JavaScript to build the frontend of an application. They work on designing and developing the layout, appearance, and behavior of web pages or mobile screens, ensuring that they are visually appealing, responsive, and intuitive to use.
Backend development, on the other hand, deals with the server-side of an application. It involves handling the business logic, data storage, and processing tasks that are necessary for the application to function properly. Backend developers work with programming languages like Python, Java, or Ruby and frameworks like Node.js or Django. They build the server infrastructure, implement APIs (Application Programming Interfaces) to communicate with the frontend, and interact with databases and external services to manage and process data.
3-tier architecture
Three-tier architecture is a software architecture pattern that divides an application into three distinct layers, each responsible for specific functionalities. This architectural approach separates the user interface, business logic, and data storage into separate layers, promoting modularity, scalability, and maintainability.
-
Presentation Layer: The presentation layer, also known as the client layer or the user interface (UI) layer, is responsible for the user interaction and interface. It focuses on displaying information to users and receiving user input. This layer includes components such as web pages, mobile screens, forms, and UI elements. It communicates with the other layers to retrieve and present data, as well as to handle user actions and inputs. The presentation layer is often implemented using technologies like HTML, CSS, JavaScript, or client-side frameworks.
-
Application Layer (Business Logic Layer): The application layer, also referred to as the business logic layer or the middle layer, contains the core functionality and business rules of the application. It processes requests from the presentation layer, performs computations, implements business logic, and interacts with data sources and external services. The application layer is responsible for validating and manipulating data, enforcing business rules, and coordinating the flow of data and operations within the application. It encapsulates the application’s logic and ensures its consistency and integrity.
-
Data Layer (Persistence Layer): The data layer, also known as the persistence layer or the backend layer, deals with data storage and retrieval. It manages the application’s data persistence and interacts with databases or other data storage mechanisms. The data layer is responsible for tasks such as storing data, querying databases, and handling data access. It provides the necessary interfaces and methods for the application layer to interact with the data, ensuring proper data management and integrity.
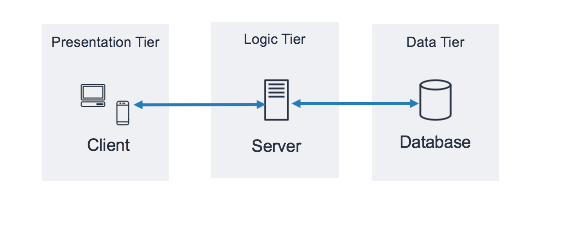
MVC design pattern
MVC stands for Model-View-Controller, which is a popular design pattern used in software development to separate the concerns of an application’s user interface, data, and control logic. The MVC design pattern provides a structured and modular approach to building applications, making them easier to understand, maintain, and modify.
-
Model: The model represents the application’s data and business logic. It encapsulates the data structure and methods for manipulating and accessing the data. The model component is responsible for managing the application’s data, performing calculations, implementing business rules, and interacting with data sources such as databases. It represents the underlying data and ensures its consistency and integrity.
-
View: The view represents the presentation of the data to the user. It is responsible for displaying information and interacting with the user interface. The view component renders the user interface elements, such as web pages, forms, or graphical interfaces, based on the data provided by the model. It focuses on the visual aspects and user experience, without having direct knowledge of the data or the business logic behind it.
-
Controller: The controller acts as the intermediary between the model and the view. It handles user input, updates the model based on the user’s actions, and updates the view accordingly. The controller receives user input from the view, processes it, and triggers the appropriate actions in the model. It also communicates changes in the model to the view, ensuring that the user interface reflects the updated data. The controller component orchestrates the flow of data and controls the interactions between the model and the view.
3-tier vs. MVC
The MVC design pattern is a design pattern that helps structure the internal components of a single application, while the 3-tier architecture is an architectural pattern that focuses on the overall organization and separation of concerns within an entire system. They can complement each other, and it is possible to implement the MVC design pattern within one or more layers of the 3-tier architecture to achieve a well-structured and modular system.
Overview of web development technologies
HTML, CSS, and JavaScript are three fundamental technologies used in web development to create and enhance websites and web applications.
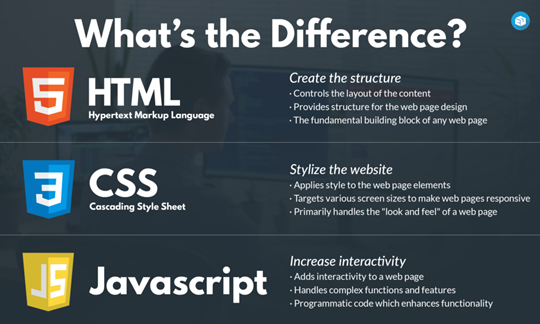
-
HTML (Hypertext Markup Language): HTML is the standard markup language used for creating the structure and content of web pages. It provides a set of predefined tags that define the elements of a webpage, such as headings, paragraphs, links, images, tables, forms, and more. HTML tags are used to structure and organize the content, allowing browsers to understand and render the page correctly. HTML is a static language, meaning it defines the structure and content of the page but doesn’t provide interactivity or dynamic behavior.
-
CSS (Cascading Style Sheets): CSS is a styling language used to control the visual appearance of HTML elements on a web page. It allows developers to define the layout, colors, fonts, spacing, and other visual aspects of the webpage. With CSS, you can create consistent and visually appealing designs by applying styles to HTML elements. CSS works by selecting HTML elements using selectors and applying specific styles or properties to those elements. It enables the separation of content and presentation, making it easier to update and maintain the styling of a website.
-
JavaScript: JavaScript is a programming language that adds interactivity, dynamic behavior, and functionality to web pages. It allows developers to create interactive features, handle events, manipulate HTML elements, perform calculations, make HTTP requests, and much more. JavaScript can be used to respond to user actions, validate form inputs, create animations, update content dynamically, and interact with APIs to fetch and manipulate data. It is a versatile language that runs on the client-side (in the user’s browser) and can also be used on the server-side (with technologies like Node.js).
Full Stack Software Engineering for Beginners
June 23rd, 2023
HTML basics: tags, structure, and semantics
HTML, which stands for Hypertext Markup Language, is the standard markup language used for creating and structuring the content of web pages. It provides a set of tags or elements that define the structure, layout, and presentation of the information displayed on a webpage.
HTML <head>
and HTML <body>
HTML documents typically include a <head>
section, which contains meta-information about the page such as the title, character encoding, and linked stylesheets or scripts. The main content of the page is usually placed within the <body>
section, which includes various elements like headings, paragraphs, lists, images, tables, forms, and more.
HTML DOM
HTML follows a hierarchical structure known as the Document Object Model (DOM), where elements can be nested inside one another to create a tree-like structure. This structure determines the layout and organization of the content on the webpage.
Each element in the document is represented as an object. This object-oriented representation of the HTML document allows developers to manipulate, access, and modify the content, structure, and style of the webpage dynamically.
HTML tags
HTML tags are the building blocks of HTML documents. They are used to mark up and define the structure, content, and presentation of the elements within a webpage. Tags are written in angle brackets < >
and come in pairs: an opening tag and a closing tag.
The opening tag denotes the start of an element and is represented by the tag name enclosed within angle brackets. For example, <p>
is the opening tag for a paragraph element.
The closing tag signifies the end of an element and consists of the same tag name as the opening tag, preceded by a forward slash. For instance, </p>
is the closing tag for a paragraph element.
Some tags, known as self-closing or void tags, do not require a closing tag and end with a forward slash before the closing angle bracket. An example of a self-closing tag is the <img>
tag used for inserting images.
HTML semantics: improving readability
HTML semantics refers to the practice of using HTML elements in a way that conveys the meaning and structure of the content within a webpage. It involves choosing appropriate HTML tags and attributes to provide clear and meaningful markup, enhancing the accessibility, maintainability, and search engine optimization of the webpage.
-
Meaningful element selection: Using the appropriate HTML tags to represent the different types of content. For example, using
<h1>
for headings,<p>
for paragraphs,<nav>
for navigation menus,<footer>
for the page footer, and so on. This helps convey the hierarchical structure and relationships of the content. -
Structuring content: Grouping related content using appropriate elements. For instance, using
<section>
to divide the content into distinct sections,<article>
for self-contained content,<header>
and<footer>
for page headers and footers, and<aside>
for sidebars or additional content. -
Accessibility: Semantic HTML aids accessibility by providing a clear and logical structure to assistive technologies such as screen readers. Properly marked-up content allows users with disabilities to navigate and understand the content more easily.
-
Search engine optimization (SEO): Search engines rely on HTML semantics to understand and index web content accurately. By using semantic markup, developers can provide better context and relevance to search engines, potentially improving the visibility and ranking of webpages in search results.
-
Maintenance and extensibility: Semantic HTML promotes maintainable and extensible code. Well-structured and meaningful markup makes it easier for developers to understand and modify the content, especially when collaborating on projects or when performing updates in the future.
-
Future-proofing: HTML semantics ensure compatibility and adaptability with evolving web standards and technologies. Semantic markup enhances the forward compatibility of webpages, making them more resilient to changes in browser behavior or new HTML versions.
Creating a simple webpage using HTML
HTML (Hypertext Markup Language) is the foundational language for creating webpages and provides the structure and content of a webpage. Using HTML alone, you can create a webpage by writing the HTML markup, including the appropriate HTML tags to structure and organize your content. For example, you can define headings with <h1>
to <h6>
tags, paragraphs with <p>
tags, images with <img>
tags, and links with <a>
tags.
Sample 1: Header and paragraph
<!DOCTYPE html>
<html>
<head>
<title>Sample 1</title>
</head>
<body>
<h1>Welcome to My Webpage</h1>
<p>This is a paragraph of text.</p>
</body>
</html>
Sample 2: Image and hyperlink
<!DOCTYPE html>
<html>
<head>
<title>Sample 2</title>
</head>
<body>
<h1>Welcome to My Webpage</h1>
<p>This is a sample with an image and a link.</p>
<img src="https://hips.hearstapps.com/hmg-prod/images/little-cute-maltipoo-puppy-royalty-free-image-1652926025.jpg?crop=0.444xw:1.00xh;0.129xw,0&resize=980:*" alt="An image">
<a href="https://www.example.com">Visit Example.com</a>
</body>
</html>
Sample 3: Table, ordered list, unordered list
<!DOCTYPE html>
<html>
<head>
<title>Sample 3</title>
</head>
<body>
<h1>Welcome to My Webpage</h1>
<h2>Table Example</h2>
<table>
<tr>
<th>Name</th>
<th>Age</th>
<th>Country</th>
</tr>
<tr>
<td>John Doe</td>
<td>30</td>
<td>USA</td>
</tr>
<tr>
<td>Jane Smith</td>
<td>25</td>
<td>Canada</td>
</tr>
</table>
<h2>Ordered List Example</h2>
<ol>
<li>First item</li>
<li>Second item</li>
<li>Third item</li>
</ol>
<h2>Unordered List Example</h2>
<ul>
<li>Red</li>
<li>Green</li>
<li>Blue</li>
</ul>
</body>
</html>
Note While HTML alone can create static webpages with text, images, links, and basic formatting, it does not handle dynamic functionality or styling.
Introduction to CSS: selectors, properties, and styling
CSS (Cascading Style Sheets) is a styling language used to describe the presentation and appearance of an HTML document. It provides a set of rules that define how HTML elements should be displayed on a webpage, including their layout, colors, fonts, sizes, and other visual properties.
CSS separates the content of a webpage (defined by HTML) from its presentation, allowing developers to control the look and feel of the webpage independently. By using CSS, you can create consistent and visually appealing designs across multiple webpages or an entire website.
CSS selectors
CSS uses selectors to target specific HTML elements on which to apply styles. Selectors can be based on element names, class names, IDs, attributes, and more. For example, you can select all paragraphs (p
), elements with a specific class (.my-class
), or elements with a specific ID (#my-id
).
- Element selector: Targets all elements of a specific type.
p { color: blue; }
In this example, the
p
selector targets all<p>
elements and sets their text color to blue. - Class selector: Targets elements with a specific class attribute.
.highlight { background-color: yellow; }
In this example, the
.highlight
selector targets all elements with the class “highlight” and applies a yellow background color. - ID selector: Targets elements with a specific ID attribute.
#header { font-size: 24px; }
In this example, the
#header
selector targets the element with the ID “header” and sets its font size to 24 pixels.
CSS properties
CSS properties define specific aspects of an element’s appearance, such as color, font-size, background-color, and margin. Each property is assigned a value that determines the desired style. For instance, color: blue;
sets the text color to blue.
CSS style rules
CSS rules consist of one or more selectors and the corresponding style declarations enclosed in curly braces { }
. Style declarations consist of property-value pairs separated by a colon :
. Multiple style rules can be defined within a CSS file or directly within an HTML document using the <style>
tag.
Cascading and Specificity
Cascading
Cascading refers to the process of combining styles from different sources (such as external stylesheets, internal styles, and inline styles) and resolving conflicts between them.
The order of precedence in cascading is as follows, from highest to lowest:
- Inline styles: Styles defined directly within the HTML element using the
style
attribute. - Internal styles: Styles defined within the
<style>
tags in the<head>
section of the HTML document. - External stylesheets: Styles defined in an external CSS file linked to the HTML document using the
<link>
tag.
When conflicting styles are encountered, the later-applied styles override the earlier ones. Inline styles have the highest precedence, followed by internal styles, and finally external stylesheets. This allows for flexibility and easy customization of individual elements.
Specificity
Specificity determines which CSS rule takes precedence when multiple rules target the same element. It is calculated based on the specificity of the selectors used in the rules.
Specificity is determined by the following factors:
- Element type selectors have the lowest specificity.
- Class selectors have a higher specificity.
- ID selectors have an even higher specificity.
- Inline styles have the highest specificity.
To calculate the specificity of a selector, each factor is assigned a numerical value. The more specific a selector is, the higher its value. For example, an ID selector has a higher value than a class selector.
When conflicting styles arise, the rule with the highest specificity is applied. If multiple rules have the same specificity, the one that appears later in the CSS file takes precedence.
p {
color: blue;
}
.highlight {
color: red;
}
#special {
color: green;
}
In this example, if we have a <p>
element with class “highlight” and ID “special”, the color applied will be green. The ID selector has the highest specificity and overrides the other selectors.
HTML with CSS
sample.html
<!DOCTYPE html>
<html>
<head>
<title>Sample HTML Page</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<h1>Welcome to Sample HTML Page</h1>
<p class="highlight">This is a paragraph with a class "highlight".</p>
<p id="special">This is a paragraph with an ID "special".</p>
</body>
</html>
styles.css
h1 {
color: blue;
}
p.highlight {
background-color: yellow;
}
#special {
font-weight: bold;
}